function rotate_image_alpha ($image, $angle, $bgcolor, $bgtransparency
{
// seen at http://www.php.net/manual/en/function.imagerotate.php
$srcw = imagesx$image);
$srch = imagesy$image);
//Normalize angle
$angle %= 360;
//Set rotate to clockwise
$angle = -$angle
if$angle == 0) {
return $image
}
// Convert the angle to radians
$theta = deg2rad ($angle);
// Standard case of rotate
if ( (abs$angle) == 90) || (abs$angle) == 270) ) {
$width = $srch
$height = $srcw
if ( ($angle == 90) || ($angle == -270) ) {
$minX = 0;
$maxX = $width
$minY = -$height+1;
$maxY = 1;
$sin = 1;
} else if ( ($angle == -90) || ($angle == 270) ) {
$minX = -$width+1;
$maxX = 1;
$minY = 0;
$maxY = $height
$sin = -1;
}
$cos = 0;
} else if (abs$angle) === 180) {
$width = $srcw
$height = $srch
$minX = -$width+1;
$maxX = 1;
$minY = -$height+1;
$maxY = 1;
$sin = 0;
$cos = -1;
} else {
$sin = sin$theta);
$cos = cos$theta);
// Calculate the width of the destination image.
$temp = array (0,
$srcw * $cos
$srch * $sin
$srcw * $cos + $srch * $sin
);
$minX = floormin$temp));
$maxX = ceilmax$temp));
$width = $maxX - $minX
// Calculate the height of the destination image.
$temp = array (0,
$srcw * $sin * -1,
$srch * $cos
$srcw * $sin * -1 + $srch * $cos
);
$minY = floormin$temp));
$maxY = ceilmax$temp));
$height = $maxY - $minY
}
$destimg = imagecreatetruecolor$width, $height);
$bgcolor = allocate_color$destimg, $bgcolor, $bgtransparency);
imagefill$destimg, 0, 0, $bgcolor);
imagesavealpha$destimg, true);
// sets all pixels in the new image
for$x$minX; $x$maxX; $x++) {
for$y$minY; $y$maxY; $y++) {
// fetch corresponding pixel from the source image
$srcX = round$x * $cos - $y * $sin);
$srcY = round$x * $sin + $y * $cos);
if$srcX >= 0 && $srcX < $srcw && $srcY >= 0 && $srcY < $srch) {
$color = imagecolorat$image, $srcX, $srcY);
} else {
$color = $bgcolor
}
imagesetpixel$destimg, $x$minX, $y$minY, $color);
}
}
return $destimg
}
eXorithm – Execute Algorithm: View / Run Algorithm rotate_image_alpha
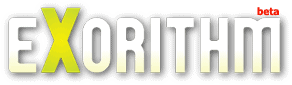